This page was generated from
regression-analysis/example_densityreg.ipynb.
Interactive online version:
.
Interactive online version:
Note
Plot a fit over a density plot
Use the densityreg
plot method to combine fits and their raw data.
This example uses artifical data to show you the capabilities of the densityreg
plot method.
[1]:
import numpy as np
import xarray as xr
import psyplot.project as psy
%matplotlib inline
%config InlineBackend.close_figures = False
First we define our data which comes from multiple realizations of the underlying equation sin(x)
[2]:
all_x = []
all_y = []
for i in range(30):
deviation = np.abs(np.random.normal())
all_x.append(np.linspace(-np.pi - deviation, np.pi + deviation))
all_y.append(np.sin(all_x[-1]) + np.random.normal(scale=0.5, size=all_x[-1].size))
x = np.concatenate(all_x)
y = np.concatenate(all_y)
ds = xr.Dataset({'x': xr.Variable(('experiment', ), x),
'y': xr.Variable(('experiment', ), y)})
ds
[2]:
<xarray.Dataset> Dimensions: (experiment: 1500) Dimensions without coordinates: experiment Data variables: x (experiment) float64 -4.74 -4.547 -4.353 ... 3.283 3.429 3.575 y (experiment) float64 0.8622 1.2 0.965 ... -1.122 -0.01563 -0.7345
This dataset now contains the two variables x
and y
. A scatter plot of the data looks like
[3]:
psy.plot.lineplot(ds, name='y', coord='x', marker='o', linewidth=0)
[3]:
psyplot.project.Project([arr0: psyplot.data.InteractiveList([ arr0: 1-dim DataArray of y, with (experiment)=(1500,), ])])
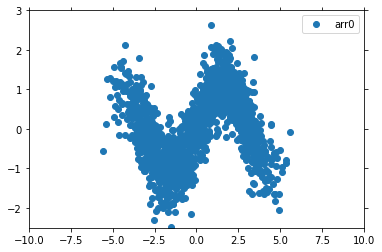
However, it is hard to see how many data points there are shown. Therefore this is a good candidate for a density
plot:
[4]:
psy.plot.density(ds, name='y', coord='x', cmap='Reds', bins=50, density='kde',
clabel='Kernel density')
[4]:
psyplot.project.Project([ arr1: 1-dim DataArray of y, with (experiment)=(1500,), ])
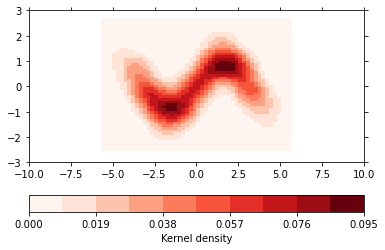
The densityreg
plot method combines this plot with a fit through the data
[5]:
psy.close('all')
psy.plot.densityreg(ds, name='y', coord='x', cmap='Reds', bins=50, density='kde',
clabel='Kernel density',
color='Blues_r', fit=lambda x, a: np.sin(a * x),
legendlabels='$\sin (%(a)1.2f * %(xname)s$)')
/home/circleci/miniconda3/envs/docs/lib/python3.8/site-packages/psy_reg/utils.py:110: RuntimeWarning: Need finite parameter boundaries for automatic initial parameter estimation!
warn("Need finite parameter boundaries for automatic initial "
[5]:
psyplot.project.Project([ arr0: 1-dim DataArray of y, with (experiment)=(1500,), ])
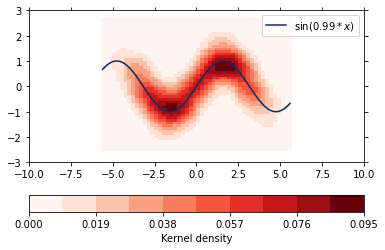
[6]:
psy.close('all')